iOS6から使えるUICollectionViewを試しました。
UICollectionViewはこういうやつです。
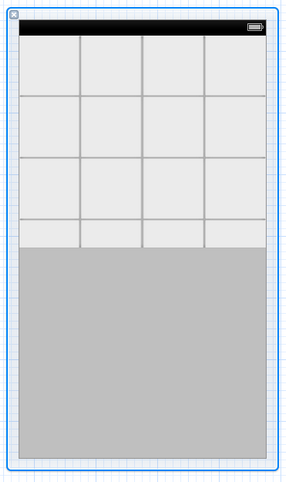
サンプルプロジェクトファイル(SampleCollectionView.zip)

ファイルはこんな感じにする。

RootViewController.h
#import <UIKit/UIKit.h>
#import "CollectionCell.h"
#import "CollectionHeaderView.h"
@interface RootViewController : UIViewController{
@private
NSMutableArray*photos;
int cellcnt;
}
@property (retain, nonatomic) IBOutlet UICollectionView *collectionView;
@end
RootViewController.m 追記部分
-(NSString*)pzero:(NSString*)s keta:(int)keta{
if (s.length>=keta) {
return s;
}
do {
s=[NSString stringWithFormat:@"0%@",s];
} while (s.length<keta);
return s;
}
-(NSString*)pzeroByInt:(int)n keta:(int)keta{
NSString*s=[NSString stringWithFormat:@"%d",n];
if (s.length>=keta) {
return s;
}
do {
s=[NSString stringWithFormat:@"0%@",s];
} while (s.length<keta);
return s;
}
-(float)getFillRatio:(CGSize)sourceSize targetSize:(CGSize)targetSize{
CGFloat widthRatio = targetSize.width / sourceSize.width;
CGFloat heightRatio = targetSize.height / sourceSize.height;
return (widthRatio > heightRatio) ? widthRatio : heightRatio;
}
-(UIImage*)getResizeImageFillCrop:(UIImage*)img size:(CGSize)_size{
CGFloat ratio=[self getFillRatio:img.size targetSize:_size];
CGSize resizedSize = CGSizeMake(roundf(img.size.width*ratio), roundf(img.size.height*ratio));
UIGraphicsBeginImageContext(resizedSize);
[img drawInRect:CGRectMake(0, 0, resizedSize.width, resizedSize.height)];
UIImage* resizedImage = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return [self getCropImage:resizedImage rect:CGRectMake((resizedImage.size.width-_size.width)*0.5, (resizedImage.size.height-_size.height)*0.5, _size.width, _size.height)];
}
-(UIImage*)getCropImage:(UIImage*)img rect:(CGRect)rect{
CGImageRef imgref=CGImageCreateWithImageInRect([img CGImage],rect);
UIImage *result=[UIImage imageWithCGImage:imgref];
CGImageRelease(imgref);
return result;
}
-(void)initCollection{
photos=[[NSMutableArray alloc]initWithCapacity:11];
int i;
for (i=0; i<23; i++) {
UIImage*img=[UIImage imageNamed:[NSString stringWithFormat:@"sc%@.png",[self pzeroByInt:i keta:2]]];
[photos addObject:[self getResizeImageFillCrop:img size:CGSizeMake(78, 78)]];
//[photos addObject:img];
}
cellcnt=[photos count];
[self.collectionView registerClass:[CollectionCell class] forCellWithReuseIdentifier:@"MY_CELL"];
UINib *headerNib = [UINib nibWithNibName:@"CollectionHeaderView" bundle:nil];
[self.collectionView registerNib:headerNib forSupplementaryViewOfKind:UICollectionElementKindSectionHeader withReuseIdentifier:@"MY_HEADER"];
}
-(void)resetCollection{
[self.collectionView reloadData];
}
- (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView {
return 1;
}
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
return [photos count];
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
CollectionCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"MY_CELL" forIndexPath:indexPath];
cell.imageview.image=[photos objectAtIndex:indexPath.item];
return cell;
}
- (UICollectionReusableView *)collectionView:(UICollectionView *)collectionView viewForSupplementaryElementOfKind:(NSString *)kind atIndexPath:(NSIndexPath *)indexPath {
CollectionHeaderView *headerView=[collectionView dequeueReusableSupplementaryViewOfKind:UICollectionElementKindSectionHeader withReuseIdentifier:@"MY_HEADER" forIndexPath:indexPath];
headerView.label.text=@"セクションヘッダー";
return headerView;
}
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout referenceSizeForHeaderInSection:(NSInteger)section {
return CGSizeMake(200, 50);
}
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath {
UIImage *image = [photos objectAtIndex:indexPath.item];
return CGSizeMake(image.size.width, image.size.height);
}
- (void)viewDidLoad
{
[super viewDidLoad];
[self initCollection];
}
RootViewController.xib

CollectionCell.h
#import <UIKit/UIKit.h>
@interface CollectionCell : UICollectionViewCell{
UIImageView*imageview;
}
@property(nonatomic,assign)UIImageView*imageview;
@end
CollectionCell.m
#import "CollectionCell.h"
@implementation CollectionCell
@synthesize imageview;
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
self.backgroundColor=[UIColor whiteColor];
UIImageView *iv=[[UIImageView alloc] initWithFrame:CGRectMake(2, 2, frame.size.width - 4, frame.size.height - 4)];
iv.autoresizingMask=18;
[self.contentView addSubview:iv];
self.imageview=iv;
}
return self;
}
/*
// Only override drawRect: if you perform custom drawing.
// An empty implementation adversely affects performance during animation.
- (void)drawRect:(CGRect)rect
{
// Drawing code
}
*/
- (void)setSelected:(BOOL)selected{
[super setSelected:selected];
if(selected){
self.backgroundColor=[UIColor cyanColor];
}else{
self.backgroundColor=[UIColor whiteColor];
}
}
@end
CollectionHeaderView.h
#import <UIKit/UIKit.h>
@interface CollectionHeaderView : UICollectionReusableView
@property (nonatomic,assign) IBOutlet UILabel *label;
@end
CollectionHeaderView.m
#import "CollectionHeaderView.h"
@implementation CollectionHeaderView
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
// Initialization code
}
return self;
}
/*
// Only override drawRect: if you perform custom drawing.
// An empty implementation adversely affects performance during animation.
- (void)drawRect:(CGRect)rect
{
// Drawing code
}
*/
@end
CollectionHeaderView.xib

便利ですね